5. Cloud Hosting#
5.1. Pre-Reading#
and
5.2. The Cloud#
The âïž is just you renting time on someone elses computer.
AWS, Azure, and Digital Ocean are all commercial cloud providers that will let you pay to rent their servers. These servers are in locations throughout the world! Organizations can also run their own private âcloud,â meaning servers that they directly control and manage.
The Shared Responsibility Model is extremely helpful for understanding the cloud:
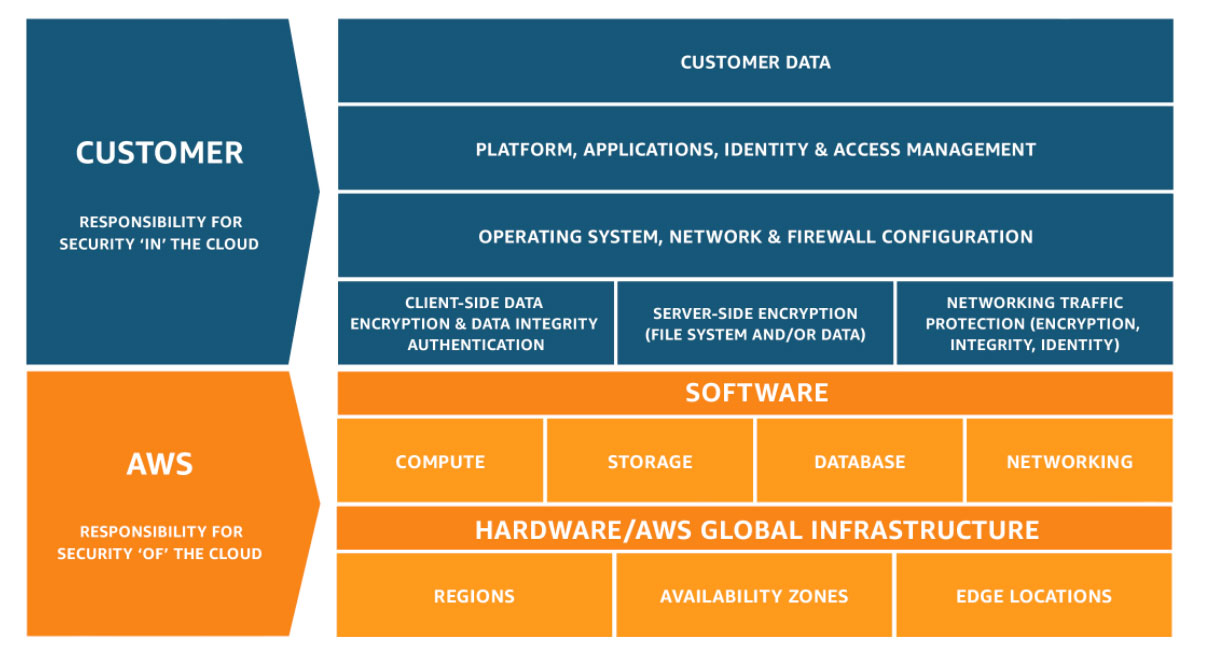
Fig. 5.1 The AWS Shared Responsibility Model details the divide between who secures the hardware and virtualization software âofâ the cloud (AWS) vs. the applications running âinâ the cloud (the customer).#
It is astonishing what cloud providers can serve you! But that dependsâŠ
On how well your manners are, and how big your pocketbook is. pic.twitter.com/rW1GyKhSpI
— Star Wars (@starwars) May 9, 2022
Cloud Resources#
This is a crazy complex space. You can get various certifications or complete free learning:
AWS Training and Certification
(AWS Certified Cloud Solutions Architect is the one to start with if you really want to get into this world.)
Note
This content is for educational purposes only. Nothing on this page constitutes an endorsement by the U.S. Government.
AWS is currently the world leader, with Microsoft Azure and Google Cloud Provider (GCP) nipping at its heals.
DigitalOcean is smaller, but more user friendly (but also less capable) and oftentimes more affordable. They also offer $200 free credit via GitHub Student.
Virtual Machines#
You can have your very own virtual machine through AWS EC2 or DigitalOcean Droplets.
As of October 2024, here is the cheapest DO Droplet:
Memory |
vCPU |
Transfer |
SSD |
$/hr |
$/mo |
---|---|---|---|---|---|
512 MiB |
1 vCPU |
500 GiB |
10 GiB |
$0.00595 |
$4.00 |
Cloud VMs are highly configurable, but a good default start is the latest LTS release of Ubuntu.
You can also pick a geographic region to deploy the VM to. The location of a data center will impact latency, price, and legal considerations.
Object Storage#
AWS Simple Scalable Storage (S3) is the de facto standard for object storage. It is object storage, meaning you have to store/fetch/delete entire files, rather than parts of a file (changing parts of a file is block storage).
S3 is great for serving static webpages and files, off-site backups, or massive datalakes!
It has a generous free tier, then $0.023 per GB per month after that for storage. But watch out, because the data transfer out can get pricy for large applications.
Security#
To be extremely brief:
Identity and Access Management (IAM) is tricky. provides fine granularity of what people and programs can and cannot read/modify/delete but because it can be tricky to get these permissions correct, organizations often over-allocate permissions. Hackers love to take advantage of that.
âSecureâ is rarely the default. (According to the shared responsibility model, thatâs your job!). For example, although S3 is now encrypted by default, it took years and several high-profile breeches for this to be the case!
Managed Services Cost Money. If you want things like automatic updates, automatic logging, and more, the cloud provide can do that for you - this will help your security posture - but it cost more cash.
5.3. Web Application Architecture#
Web applications are frequently described in terms of âfront endâ and âback end.â In this class we are exclusively concerned with the back end.
The front end is the pretty website that you see. It is a collection of HTML, CSS, and JavaScript. Your web browser executes and renders the front end code.
The back end is the part most people never see: the logic and databases that drive the application. This can be written in Go, Python, Rust, Kotlin, or many other languages.
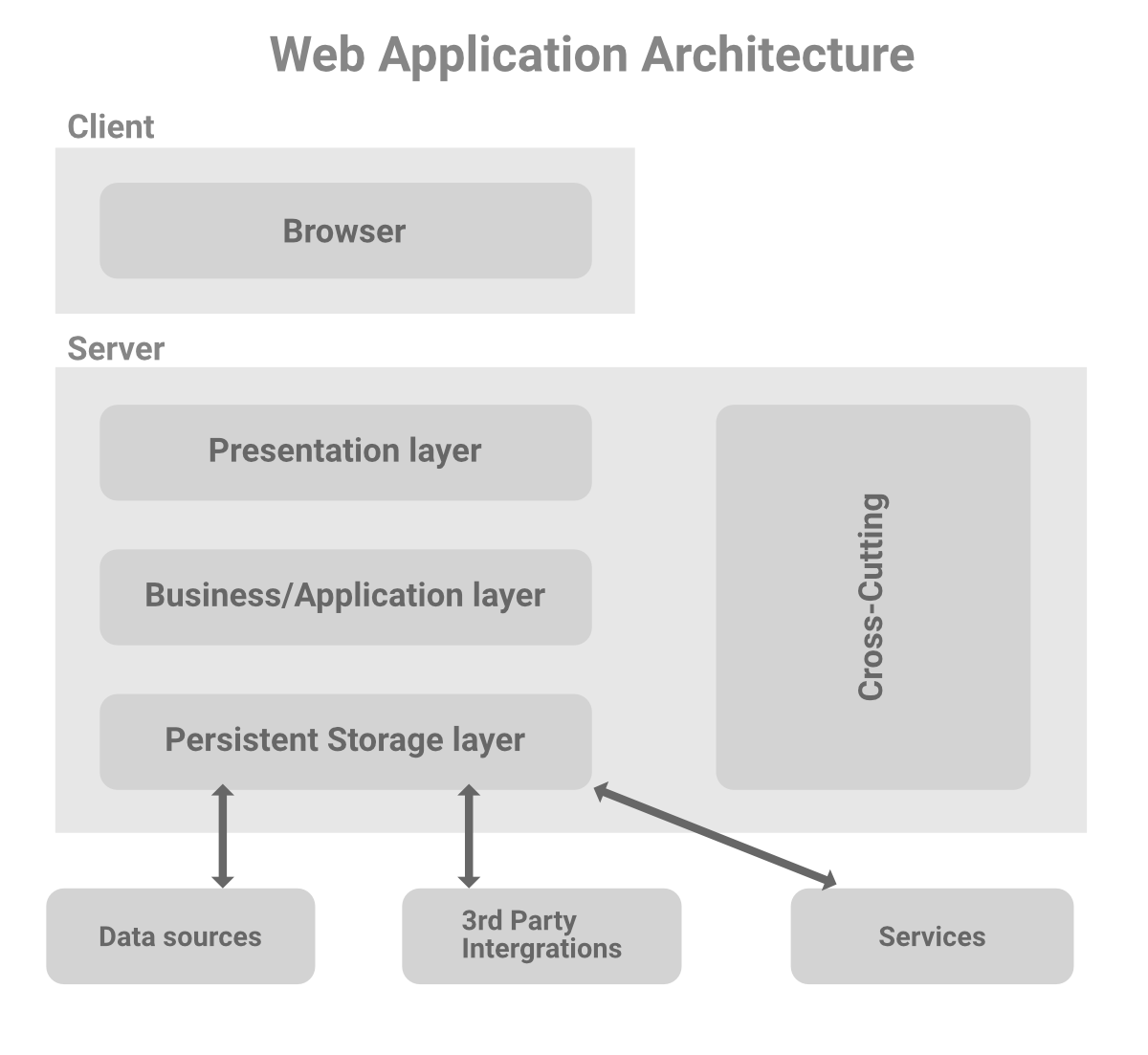
Fig. 5.2 The common Web Application Three Tier Architecture. See GeeksForGeeks: Web Applications for Beginners for more information.#
Databases are beyond the scope of this course. As a result, the apps we build will be stateless. They will focus on providing inference via a pre-trained model.
APIs#
Application Program Interfaces (APIs) are a programmatic way to interact with a web application.
There are many types, but we will use HTTP RESTful APIs in this course.
Representational State Transfer (REST) is a software architecture that imposes conditions on how an API should work. These APIs are stateless, meaning each request contains authentication tokens and other information and is isolated from other requests. This is in contrast to keeping a long-lived connection open.
Our APIs will primarily be HTTP GET requests - which get information from the server - or POST requests - which submit data to be processed.
As an example GET request, you can fetch your username from GitHub!
Use the curl
command (which makes a GET request by default):
# Replace octocat with your GitHub username
curl https://api.github.com/users/octocat
FastAPI#
A popular Python API Framework is FastAPI. Two big reasons to use it:
The documentation is a pleasure to read
It supports type annotations in path parameters and return types
FastAPI will use this return type to:
Validate the returned data.
If the data is invalid (e.g. you are missing a field), it means that your app code is broken, not returning what it should, and it will return a server error instead of returning incorrect data. This way you and your clients can be certain that they will receive the data and the data shape expected.
Add a JSON Schema for the response, in the OpenAPI path operation.
This will be used by the automatic docs.
It will also be used by automatic client code generation tools.
But most importantly:
It will limit and filter the output data to what is defined in the return type.
This is particularly important for security
5.4. Next Steps: FastAPI Demo#
We will run a simple FastAPI example!